Task 1 and 2: Pokemon EDA
Contents
Task 1 and 2: Pokemon EDA#
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
Task 1: Setting the Aesthetics for the plots#
1.1: Set the Seaborn figure theme and scale up the text in the figures#
There are five preset Seaborn themes: darkgrid
, whitegrid
, dark
, white
, and ticks
.
They are each suited to different applications and personal preferences.
You can see what they look like here.
Hint: You will need to use the font_scale
property of the set_theme()
function in Seaborn.
# Your Solution Here
Once you are able to set the theme, you will see all plots in this Jupyter Notebook update using the same theme.
Remember to copy this code above to your other Jupyter Notebooks as well!!
Task 2: Exploratory Data Analysis#
In this section of the task, you will be performing EDA on a given dataset with a goal to be able to describe it.
2.1. Describe your dataset#
Consider (and keep in mind) the following questions to guide you in your exploration:
Who: Which company/agency/organization provided this data?
What: What is in your data?
When: When was your data collected (for example, for which years)?
Why: What is the purpose of your dataset? Is it for transparency/accountability, public interest, fun, learning, etc…
How: How was your data collected? Was it a human collecting the data? Historical records digitized? Server logs?
Hint: The pokemon dataset is from this Kaggle page.
Hint: You probably will not need more than 250 words to describe your dataset. All the questions above do not need to be answered, it’s more to guide your exploration and think a little bit about the context of your data. It is also possible you will not know the answers to some of the questions above, that is FINE - data scientists are often faced with the challenge of analyzing data from unknown sources. Do your best, acknowledge the limitations of your data as well as your understanding of it. Also, make it clear what you’re speculating about. For example, “I speculate that the {…column_name…} column must be related to {….} because {….}.”
# Your Dataset Description here (convert this to a markdown cell and write some text)
2.2. Load the dataset from a file, or URL#
This needs to be a pandas dataframe. Remember that others may be running your jupyter notebook so it’s important that the data is accessible to them.
General advice: If your dataset isn’t accessible as a URL, make sure to commit it into your repo. If your dataset is too large to commit (>100 MB), and it’s not possible to get a URL to it, you should contact your instructor for advice.
For this question, luckily the dataset is available as a URL. You can use this URL to load the data: https://github.com/firasm/bits/raw/master/pokemon.csv
# Your solution here
2.3. Explore your dataset#
Which of your columns are interesting/relevant? Remember to take some notes on your observations, you’ll need them for the next EDA step (initial thoughts).
2.3.1: You should start with df.describe().T
#
See [linked documentation]((https://pandas.pydata.org/pandas-docs/stable/reference/api/pandas.DataFrame.describe.html) for the use of include
/exclude
to look at numerical and categorical data.
# Your solution to output `df.describe.T` for numerical columns:
# Your solution to output `df.describe.T` for categorical columns:
2.3.2 Let’s try pandas_profiling
now.#
Hint: To install the pandas_profiling
package, you’ll need to use conda
:
conda install -c conda-forge pandas-profiling
To use Pandas Profiling:
import pandas_profiling
# To use pandas_profiling, run this command:
pandas_profiling.ProfileReport(df)
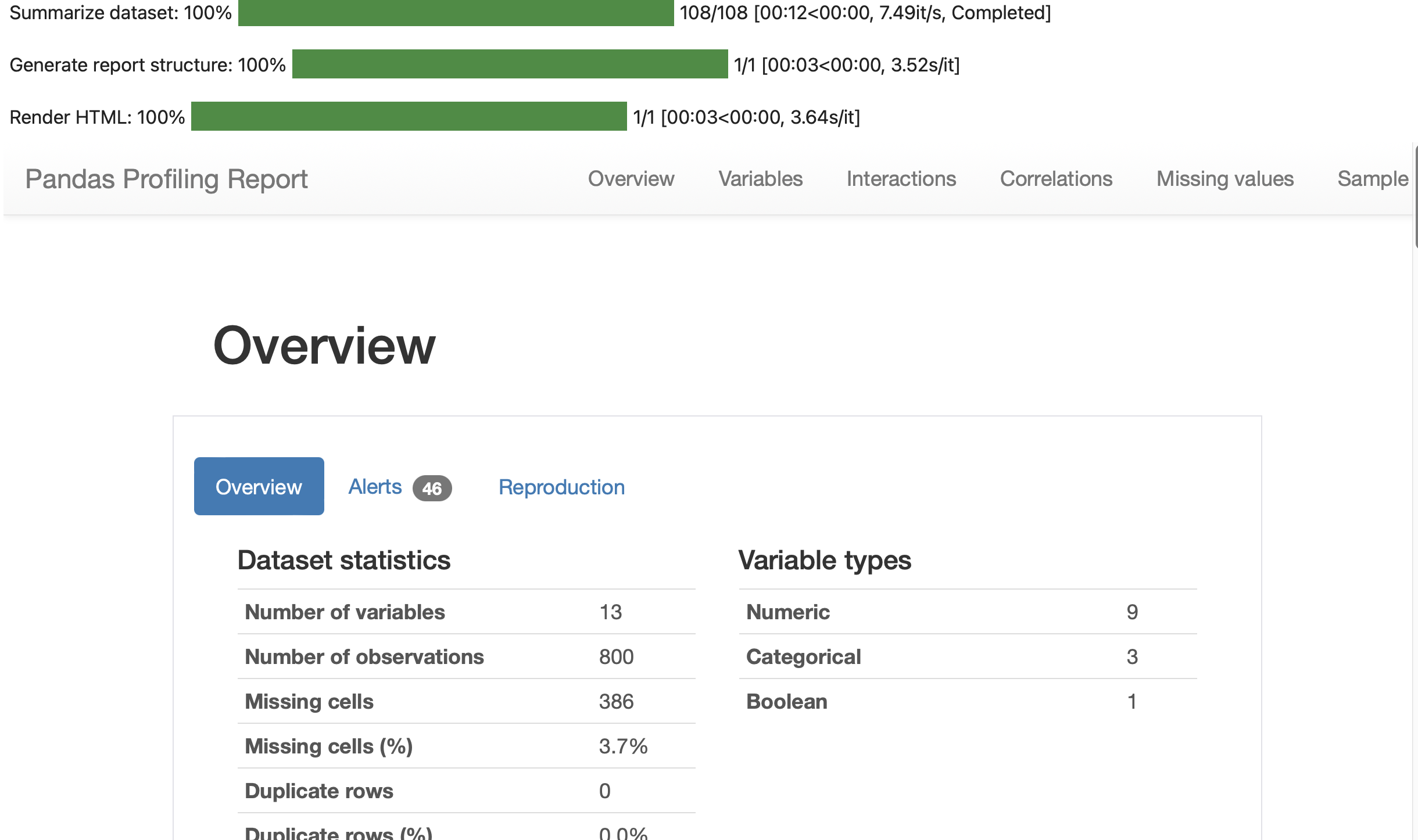
# Your solution for `pandas_profiling`
2.4. Initial Thoughts#
2.4.1. Use this section to record your observations.#
Does anything jump out at you as surprising or particularly interesting?
Where do you think you’ll go with exploring this dataset? Feel free to take notes in this section and use it as a scratch pad.
Any content in this area will only be marked for effort and completeness.
# Your observations here:#
Obs 1
Obs 2
…
2.5. Wrangling#
The next step is to wrangle your data based on your initial explorations. Normally, by this point, you have some idea of what your research question will be, and that will help you narrow and focus your dataset.
In this lab, we will guide you through some wrangling tasks with this dataset.
2.5.1. Drop the ‘Generation’, ‘Sp. Atk’, ‘Sp. Def’, ‘Total’, and the ‘#’ columns#
# Your solution here
2.5.2. Drop any NaN values in HP, Attack, Defense, Speed#
# Your solution here
2.5.3. Reset the index to get a new index without missing values#
# Your solution here
2.5.4. A new column was added called index
; remove it.#
# Your solution here
2.5.5. Calculate a new column called “Weighted Score” that computes an aggregate score comprising:#
20% ‘HP’
40% ‘Attack’
30% ‘Defense’
10% ‘Speed’
# Your solution here
2.6. Research questions#
2.6.1 Come up with at least two research questions about your dataset that will require data visualizations to help answer.#
Recall that for this purpose, you should only aim for “Descriptive” or “Exploratory” research questions.
Hint1: You are welcome to calculate any columns that you think might be useful to answer the question (or re-add dropped columns like ‘Generation’, ‘Sp. Atk’, ‘Sp. Def’.*
Hint2: Try not to overthink this; this is a toy dataset about Pokémon, you’re not going to solve climate change or cure world hunger. Focus your research questions on the various Pokémon attributes, and the types.
# Your solution here:#
1. Sample Research Question: Which Pokemon Types are the best, as determined by the Weighted Score?
2. Your RQ 1:
3. Your RQ 2:
2.7. Save your dataset#
Here, using the pandas.DataFrame.to_csv function, save your dataframe to be used in other tasks by naming it task2.csv in the data directory.
# Your solution here.