Class 4A: Introduction to Programming in Python
Contents
Class 4A: Introduction to Programming in Python#
We will begin at 3:30 PM! Until then, feel free to use the chat to socialize, and enjoy the music!
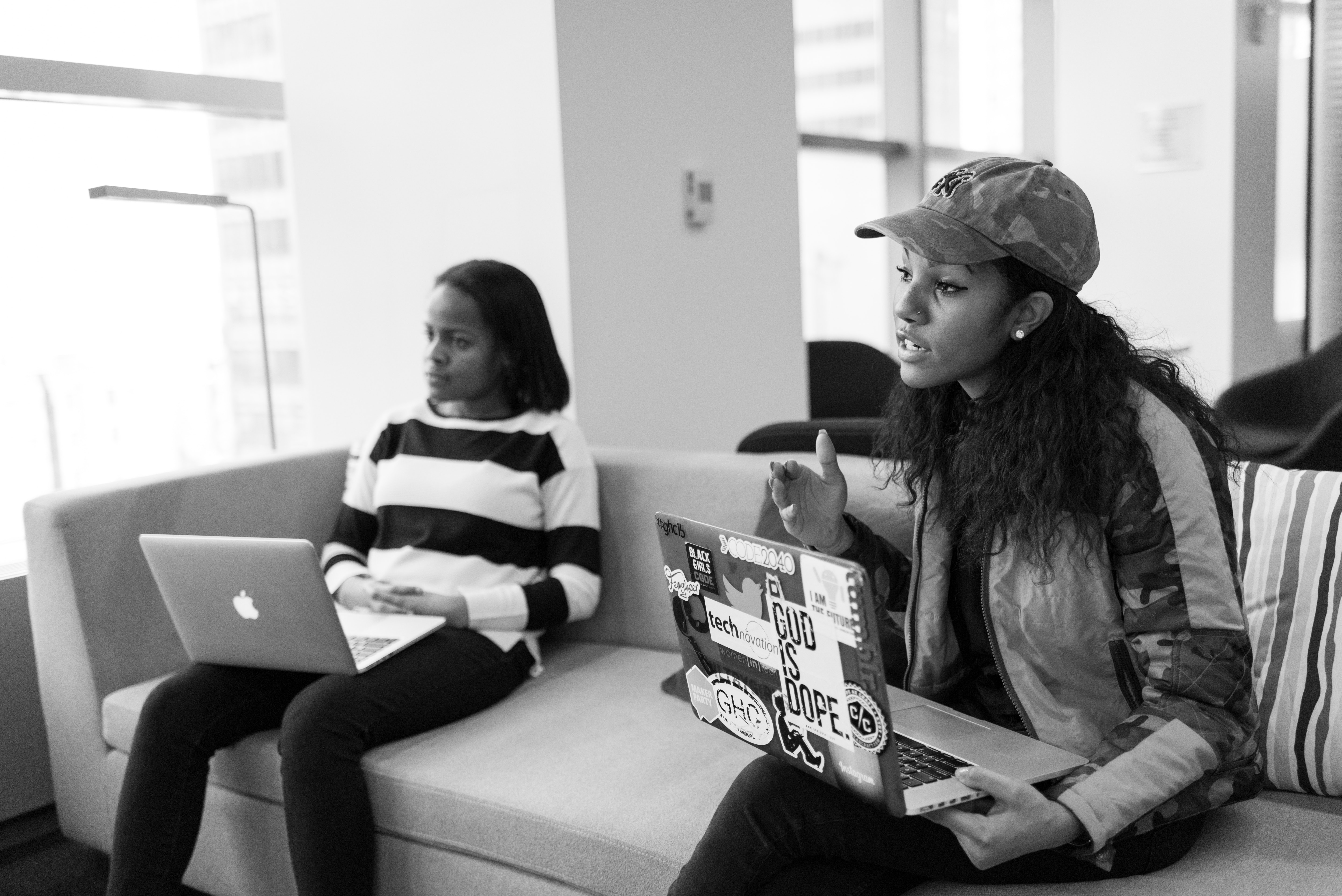
Firas Moosvi
Class Outline:#
Announcements
Lab 1 feedback has been released
Demo of doing a Resubmission Request
Reminder: Thursday class is Test 1!
Contents: Git, Terminal, Markdown, General installation info
Reminder: Friday is National Day of Truth and Reconciliation
Friday Labs are cancelled, go to another one!
1st hour - Introduction to Python
Announcements (2 mins)
Introduction (3 mins)
Writing and Running Code (15 min)
Interpreting Code (15 min)
Review and Recap (5 min)
Digging Deeper into Python syntax
Basic datatypes (15 min)
Lists and tuples (15 min)
String methods (5 min)
Dictionaries (10 min)
Conditionals (10 min)
Learning Objectives#
Look at some lines of code and predict what the output will be.
Convert an English sentence into code.
Recognize the order specific lines of code need to be run to get the desired output.
Imagine how programming can be useful to your life!
Part 1: Introduction (5 mins)#
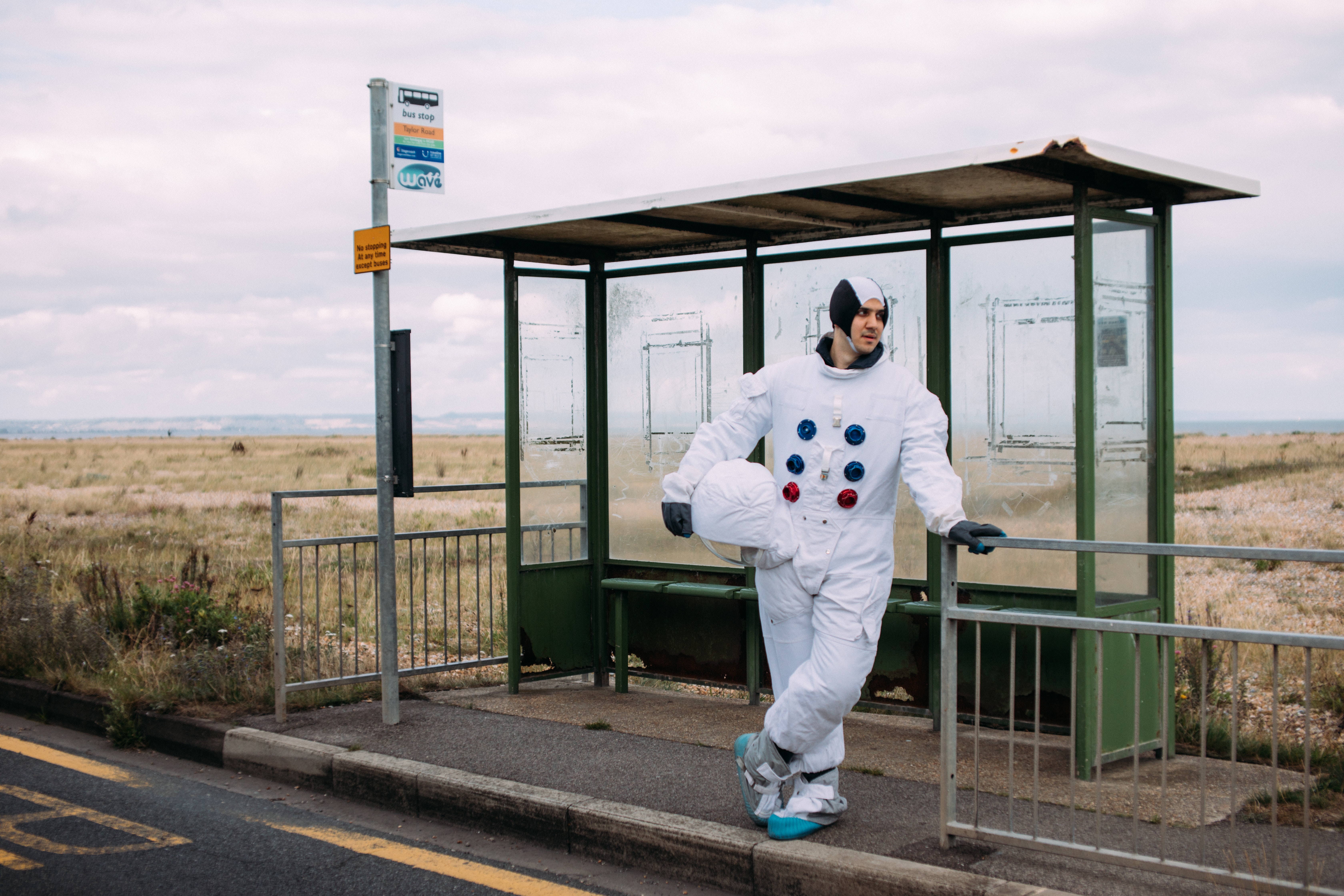
Part 2: Writing and Running code (15 mins)#
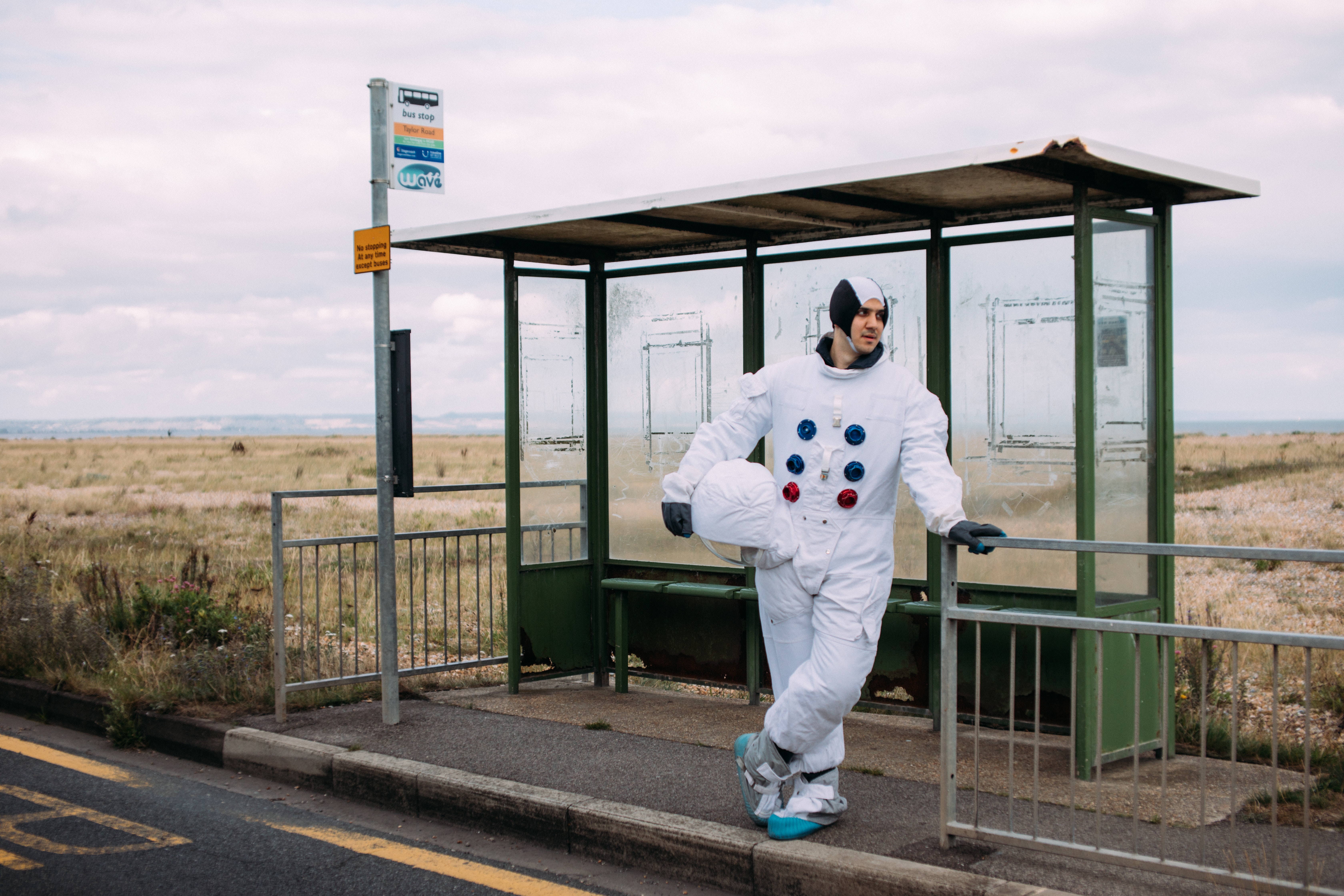
Using Python to do Math#
Task |
Symbol |
---|---|
Addition |
|
Subtraction |
|
Multiplication |
|
Division |
|
Square, cube, … |
|
Squareroot, Cuberoot, … |
|
Trigonometry (sin,cos,tan) |
Later… |
Demo (side-by-side)#
Addition#
4 + 5 + 2
11
# Subtraction
4 - 5
-1
# Multiplication
4 * 5
20
# Division
11 / 2
5.5
# Square, cube, ...
2**4
16
# Squareroot, Cuberoot, ...
9 ** (1 / 3)
2.080083823051904
Assigning numbers to variables#
You can assign numbers to a “variable”.
You can think of a variable as a “container” that represents what you assigned to it
There are some rules about “valid” names for variables, we’ll talk about the details later
Rule 1: Can’t start the name of a variable with a number!
General guideline for now: just use a combination of words and numbers
Demo (side-by-side)#
# Two numbers
num1 = 40
num2 = 8
print(num1, num2)
40 8
# Multiply numbers together
num1 * num2
320
Assigning words to variables#
You can also assign words and sentences to variables!
Surround anything that’s not a number with double quotes ” and “
mysentence = (
"This is a whole sentence with a list of sports, swimming, tennis, badminton"
)
print(mysentence)
This is a whole sentence with a list of sports, swimming, tennis, badminton
Using Python to work with words#
Python has some nifty “functions” to work with words and sentences.
Here’s a table summarizing some interesting ones, we’ll keep adding to this as the term goes on.
Task |
Function |
---|---|
Make everything upper-case |
|
Make everything lower-case |
|
Capitalize first letter of every word |
|
Count letters or sequences |
|
Demo (side-by-side)#
mysentence
'This is a whole sentence with a list of sports, swimming, tennis, badminton'
# split on a comma
mysentence.split(",")
['This is a whole sentence with a list of sports',
' swimming',
' tennis',
' badminton']
# make upper case
mysentence.upper()
'THIS IS A WHOLE SENTENCE WITH A LIST OF SPORTS, SWIMMING, TENNIS, BADMINTON'
# make lower case
mysentence.lower()
'this is a whole sentence with a list of sports, swimming, tennis, badminton'
# count the times "i" occurs
mysentence.count("i")
8
# count two characters: hi
mysentence.count("hi")
1
mysentence2 = " Hello. World ..... "
mysentence2
' Hello. World ..... '
mysentence2.strip(" ")
'Hello. World .....'
mysentence2.replace(" ", "^")
'^^^^^Hello.^^^^^World^.....^^^^^'
Part 3: Interpreting code (15 mins)#
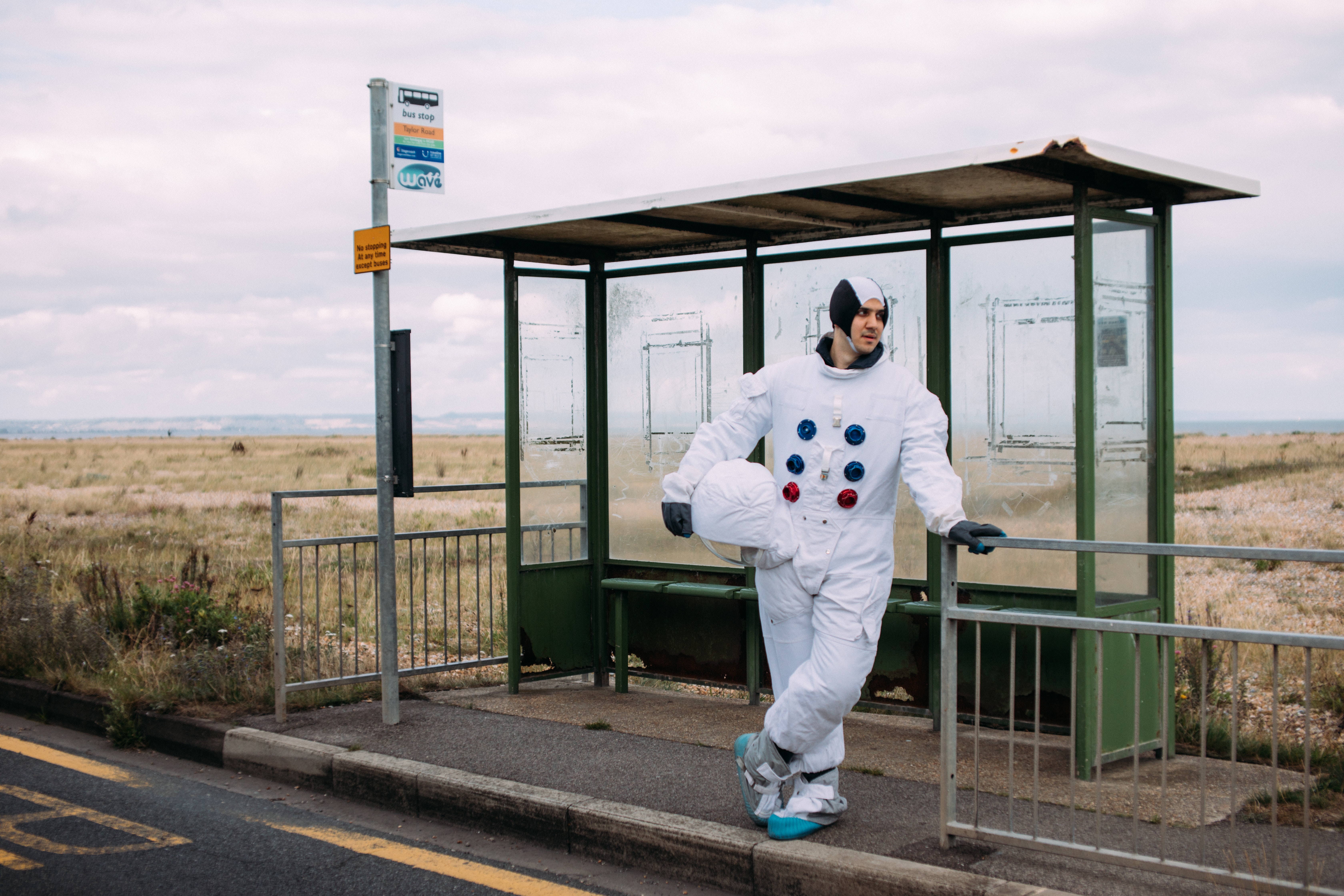
Q1: Interpret Code#
Look at the following code chunk, can you predict what the output will be?
some_numbers = [1, 50, 40, 75, 400, 1000]
for i in some_numbers:
print(i*5)
A. Prints 6 random numbers.
B. Prints the 6 numbers in some_numbers
.
C. Prints the 6 numbers in some_numbers
multiplied by 5.
D. I don’t know.
# Let's try it!
some_numbers = [1, 50, 40, 75, 400, 1000]
for i in some_numbers:
print(i * 5)
5
250
200
375
2000
5000
Q2: Interpret Code#
Look at the following code chunk, can you predict what the output will be?
some_numbers = [1, 50, 40, 75, 400, 1000]
for i in some_numbers:
if i > 50:
print(i/5)
else:
print(i)
A. Prints the 6 numbers in some_numbers
.
B. Prints the number in some_numbers
if it is less than 50, otherwise prints the number divided by 5.
C. Prints the number in some_numbers
if it is greater than 50, otherwise prints the number divided by 5.
D. I don’t know.
# Let's try it!
some_numbers = [1, 50, 40, 75, 400, 1000]
for i in some_numbers:
if i > 50:
print(i / 5)
else:
print(i)
1
50
40
15.0
80.0
200.0
Q3: Interpret Code#
Look at the following code chunk, can you predict what the output will be?
some_numbers = [1, 50, 40, 75, 400, 1000]
def process_number(number):
return (number**2)/10
for i in some_numbers:
if i > 50:
print(process_number(i))
A. Prints the number in some_numbers
if it is greater than 50, otherwise prints nothing.
B. Prints the output of the process_number()
function applied to some_numbers
.
C. Prints the output of the process_number()
function if the original number is greater than 50, otherwise prints nothing.
D. I don’t know.
# Let's try it!
some_numbers = [1, 50, 40, 75, 400, 1000]
def process_number(number):
return (number**2) / 10
for i in some_numbers:
if i > 50:
print(process_number(i))
562.5
16000.0
100000.0
Q4: Order matters!#
Suppose you are asked to complete the following operation:
Take the number 5, square it, subtract 2, and then multiply the result by 10
Does the order of the operations you do matter? Yes!
# Let's try it:
((5**2) - 2) * 10
230
# Here is the same operation as above but in multiple lines
number = 5
number = number**2
number = number - 2
number = number * 10
print(number)
230
Q5: Parson’s problem#
A Parson’s Problem is one where you are given all the lines of code to solve the problem, but they are jumbled and it’s up to you to get the right order.
A student would like to get this as the final output of some code that they are writing:
3 is smaller than, or equal to 10.
4 is smaller than, or equal to 10.
5 is smaller than, or equal to 10.
6 is smaller than, or equal to 10.
7 is smaller than, or equal to 10.
8 is smaller than, or equal to 10.
9 is smaller than, or equal to 10.
10 is smaller than, or equal to 10.
11 is bigger than 10!
12 is bigger than 10!
13 is bigger than 10!
14 is bigger than 10!
15 is bigger than 10!
Here are ALL the lines of code they will need to use, but they are scrambled in the wrong order. Can you produce the desired output?
Hint: Pay attention to the indents!
my_numbers = [3,4,5,6,7,8,9,10,11,12,13,14,15]
for i in my_numbers:
if i > 10:
print(i,'is smaller than, or equal to 10.')
else:
print(i,'is bigger than 10!')
my_numbers = [3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15]
for i in my_numbers:
if i > 10:
print(i, "is bigger than 10!")
else:
print(i, "is smaller than, or equal to 10.")
3 is smaller than, or equal to 10.
4 is smaller than, or equal to 10.
5 is smaller than, or equal to 10.
6 is smaller than, or equal to 10.
7 is smaller than, or equal to 10.
8 is smaller than, or equal to 10.
9 is smaller than, or equal to 10.
10 is smaller than, or equal to 10.
11 is bigger than 10!
12 is bigger than 10!
13 is bigger than 10!
14 is bigger than 10!
15 is bigger than 10!
Congratulations!!#
You have just shown that you can program!
Over 75% of the course programming content will be focused on details of the things you’ve seen above:
Numbers and Strings
Loops and Conditionals
Functions
If you followed along with most of what we covered, you’re in good shape for this course
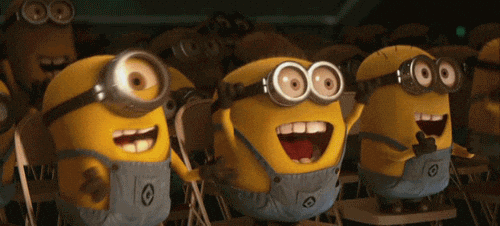
Break#
Class 4B: Introduction to Programming in Python#
Basic datatypes#
A value is a piece of data that a computer program works with such as a number or text.
There are different types of values:
42
is an integer and"Hello!"
is a string.A variable is a name that refers to a value.
In mathematics and statistics, we usually use variables names like \(x\) and \(y\).
In Python, we can use any word as a variable name (as long as it starts with a letter and is not a reserved word in Python such as
for
,while
,class
,lambda
,import
, if, else, etc.).
And we use the assignment operator
=
to assign a value to a variable.
See the Python 3 documentation for a summary of the standard built-in Python datatypes. See Think Python (Chapter 2) for a discussion of variables, expressions and statements in Python.
Common built-in Python data types#
English name |
Type name |
Description |
Example |
---|---|---|---|
integer |
|
positive/negative whole numbers |
|
floating point number |
|
real number in decimal form |
|
boolean |
|
true or false |
|
string |
|
text |
|
list |
|
a collection of objects - mutable & ordered |
|
tuple |
|
a collection of objects - immutable & ordered |
|
dictionary |
|
mapping of key-value pairs |
|
none |
|
represents no value |
|
Numeric Types#
x = 45
print(x)
45
type(x)
int
print(x)
45
x # in Jupyter we don't need to explicitly print for the last line of a cell
45
pi = 3.14159
print(pi)
3.14159
type(pi)
float
Arithmetic Operators#
The syntax for the arithmetic operators are:
Operator |
Description |
---|---|
|
addition |
|
subtraction |
|
multiplication |
|
division |
|
exponentiation |
|
integer division |
|
modulo |
Let’s apply these operators to numeric types and observe the results.
1 + 2 + 3 + 4 + 5
15
0.1 + 0.2
0.30000000000000004
Tip
Note From Firas: This is floating point arithmetic. For an explanation of what’s going on, see this tutorial.
2 * 3.14159
6.28318
2**10
1024
type(2**10)
int
2.0**10
1024.0
int_2 = 2
float_2 = 2.0
float_2_again = 2.0
101 / 2
50.5
101 // 2 # "integer division" - always rounds down
50
101 % 2 # "101 mod 2", or the remainder when 101 is divided by 2
1
None#
NoneType
is its own type in Python.It only has one possible value,
None
x = None
print(x)
None
type(x)
NoneType
You may have seen similar things in other languages, like null
in Java, etc.
Strings#
Text is stored as a datatype called a string.
We think of a string as a sequence of characters.
We write strings as characters enclosed with either:
single quotes, e.g.,
'Hello'
double quotes, e.g.,
"Goodbye"
triple single quotes, e.g.,
'''Yesterday'''
triple double quotes, e.g.,
"""Tomorrow"""
my_name = "Firas Moosvi"
print(my_name)
Firas Moosvi
type(my_name)
str
course = "COSC 301"
print(my_name, course)
Firas Moosvi COSC 301
print(my_name + " --------------> " + course)
Firas Moosvi --------------> COSC 301
type(course)
str
If the string contains a quotation or apostrophe, we can use double quotes or triple quotes to define the string.
"It's a rainy day cars'."
"It's a rainy day cars'."
sentence = "It's a rainy day."
print(sentence)
It's a rainy day.
type(sentence)
str
saying = '''They say:
"It's a rainy day!"'''
print(saying)
They say:
"It's a rainy day!"
Boolean#
The Boolean (
bool
) type has two values:True
andFalse
.
the_truth = True
print(the_truth)
True
type(the_truth)
bool
lies = False
print(lies)
False
type(lies)
bool
Comparison Operators#
Compare objects using comparison operators. The result is a Boolean value.
Operator |
Description |
---|---|
|
is |
|
is |
|
is |
|
is |
|
is |
|
is |
|
is |
2 < 3
True
"Data Science" != "Deep Learning"
True
2 == "2"
False
2 == 2.00000000000000005
True
Operators on Boolean values.
Operator |
Description |
---|---|
|
are |
|
is at least one of |
|
is |
True and True
True
True and False
False
False or False
False
# True and True
("Python 2" != "Python 3") and (2 <= 3)
True
not True
False
not not not not True
True
Casting#
Sometimes (but rarely) we need to explicitly cast a value from one type to another.
Python tries to do something reasonable, or throws an error if it has no ideas.
x = int(5.0)
x
5
type(x)
int
x = str(5.0)
x
'5.0'
type(x)
str
str(5.0) == 5.0
False
int(5.3)
5
## RISE settings
from IPython.display import IFrame
from traitlets.config.manager import BaseJSONConfigManager
from pathlib import Path
path = Path.home() / ".jupyter" / "nbconfig"
cm = BaseJSONConfigManager(config_dir=str(path))
tmp = cm.update(
"rise",
{
"theme": "sky", # blood is dark, nice
"transition": "fade",
"start_slideshow_at": "selected",
"autolaunch": False,
"width": "100%",
"height": "100%",
"header": "",
"footer": "",
"scroll": True,
"enable_chalkboard": True,
"slideNumber": True,
"center": False,
"controlsLayout": "edges",
"slideNumber": True,
"hash": True,
},
)