Task 3 - Creating Functions
Contents
Task 3 - Creating Functions#
In your projects, you may need to create your own functions to do some complex calculations (beyond mean, median etc…). In this question you will write a function to find the roots of a quadratic equation.
To refresh your memory from high school math, a quadratic function looks like this:
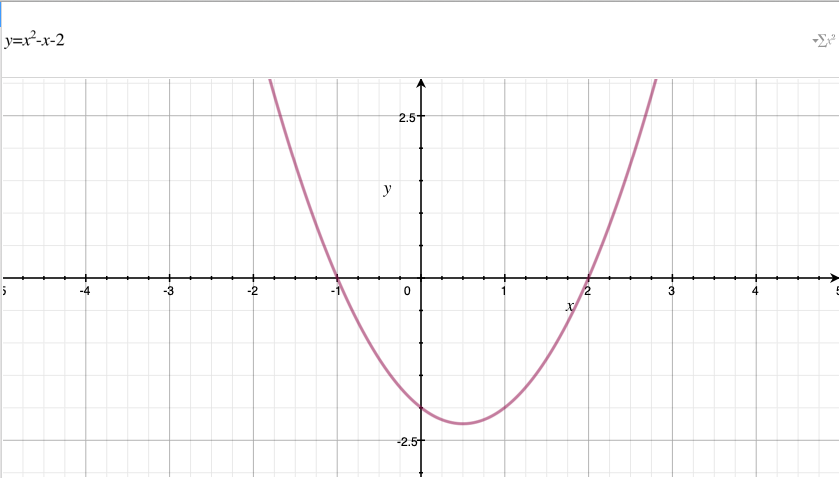
Given the standard form of a quadratic equation,
Write a python function that calculates and prints all possible real (i.e. non-complex) answers for
Check your function by running it on some test data points:
solution(1,1,1)
,solution(1,0,-4)
,solution(1,2,1)
.
Sample Output#
–> For a=1, b=1, and c=1:
The equation does not have any real solution
–> For a=1, b=0, and c=-4:
x1 = 2.0 and x2 = -2.0
–> For a=1, b=2, and c=1:
x = -1.0
#add your import statements here
def solution(a, b, c):
# Your solution here
Cell In[2], line 2
# Your solution here
^
SyntaxError: incomplete input
solution(1,1,1)
solution(1,0,-4)
solution(1,2,1)