Class 3A: Introduction to Programming in Python#
We will begin soon! Until then, feel free to use the chat to socialize, and enjoy the music!
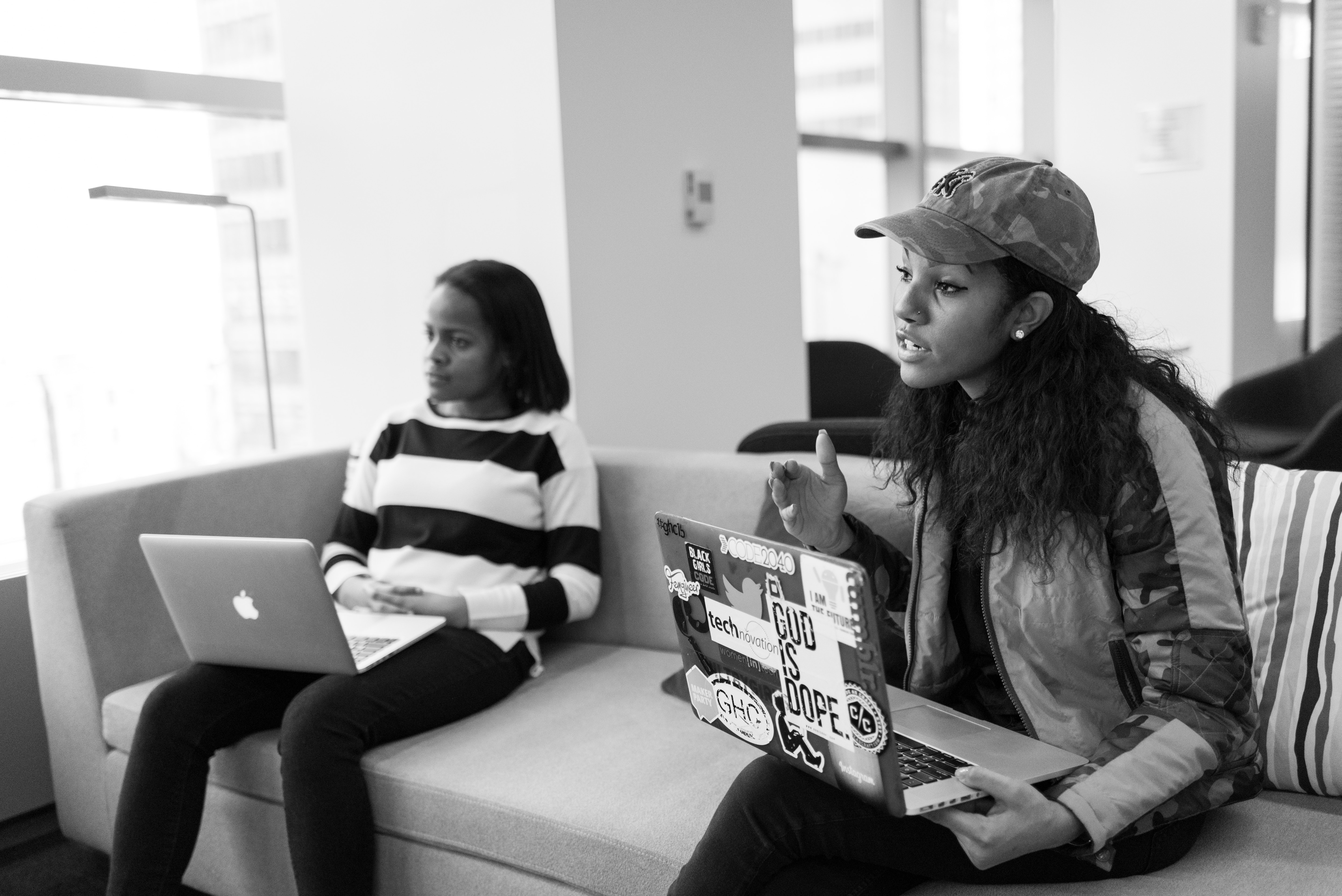
Class Outline:#
Announcements
Reminder: Next Wednesday’s class is Test 1!
Contents: Git, Terminal, Markdown, General installation info
1st hour - Introduction to Python
Announcements (2 mins)
Introduction (3 mins)
Writing and Running Code (15 min)
Interpreting Code (15 min)
Review and Recap (5 min)
Digging Deeper into Python syntax
Basic datatypes (15 min)
Lists and tuples (15 min)
String methods (5 min)
Dictionaries (10 min)
Conditionals (10 min)
Learning Objectives#
Look at some lines of code and predict what the output will be.
Convert an English sentence into code.
Recognize the order specific lines of code need to be run to get the desired output.
Imagine how programming can be useful to your life!
Part 1: Introduction (5 mins)#
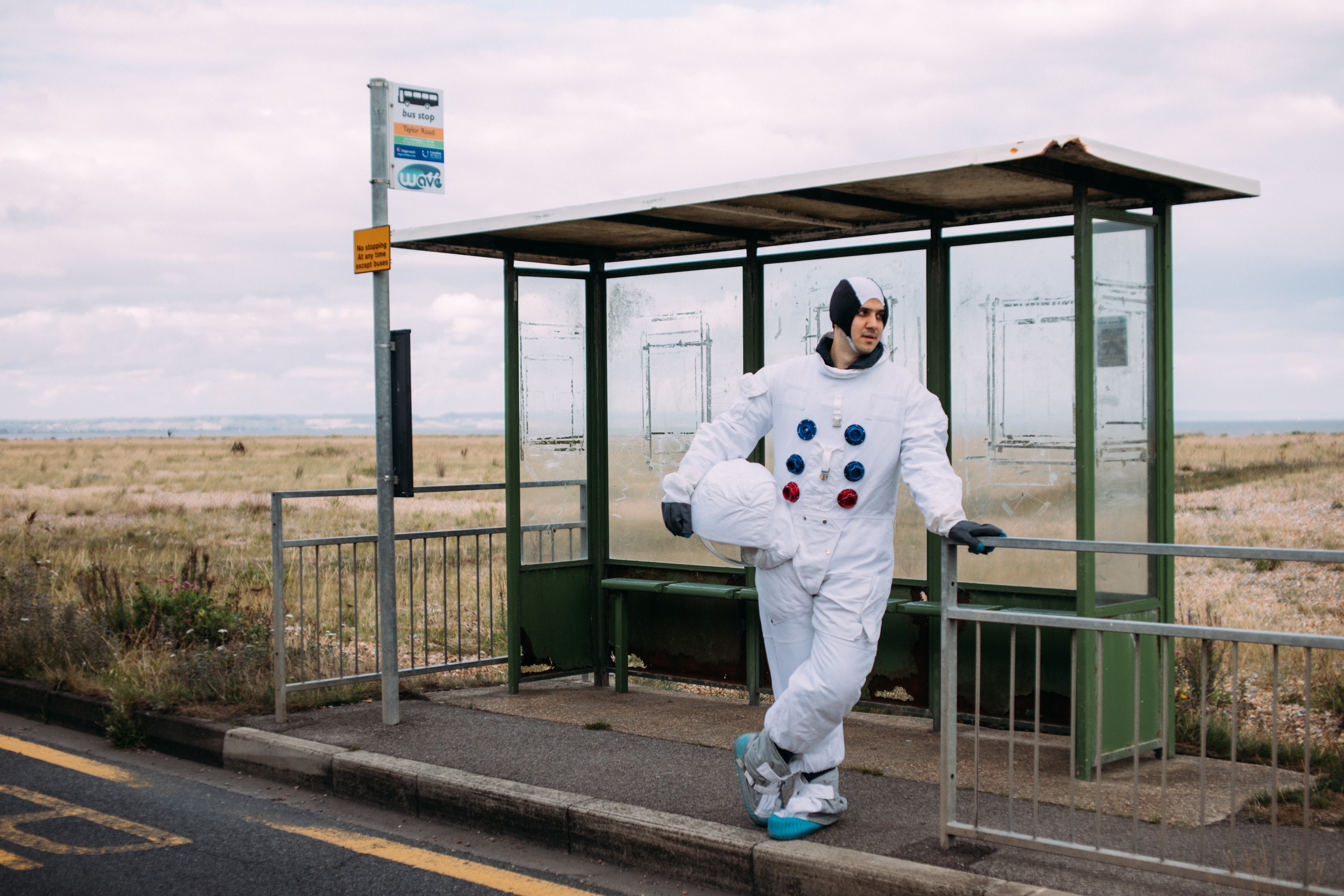
Note: Some images may look slightly different than yours as the software gets more polished and icons/user interfaces change, but this should give you a general gist of the essential things.
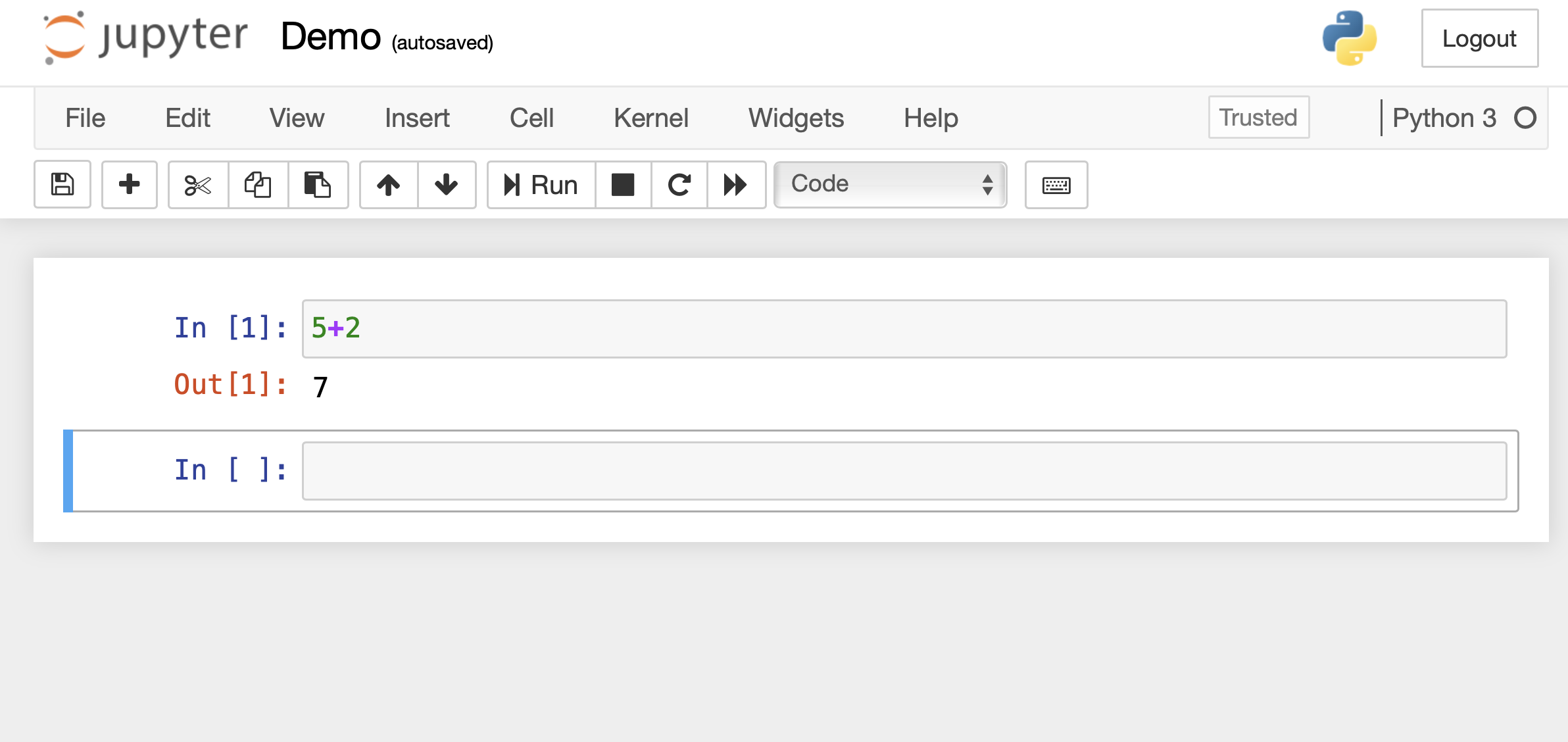
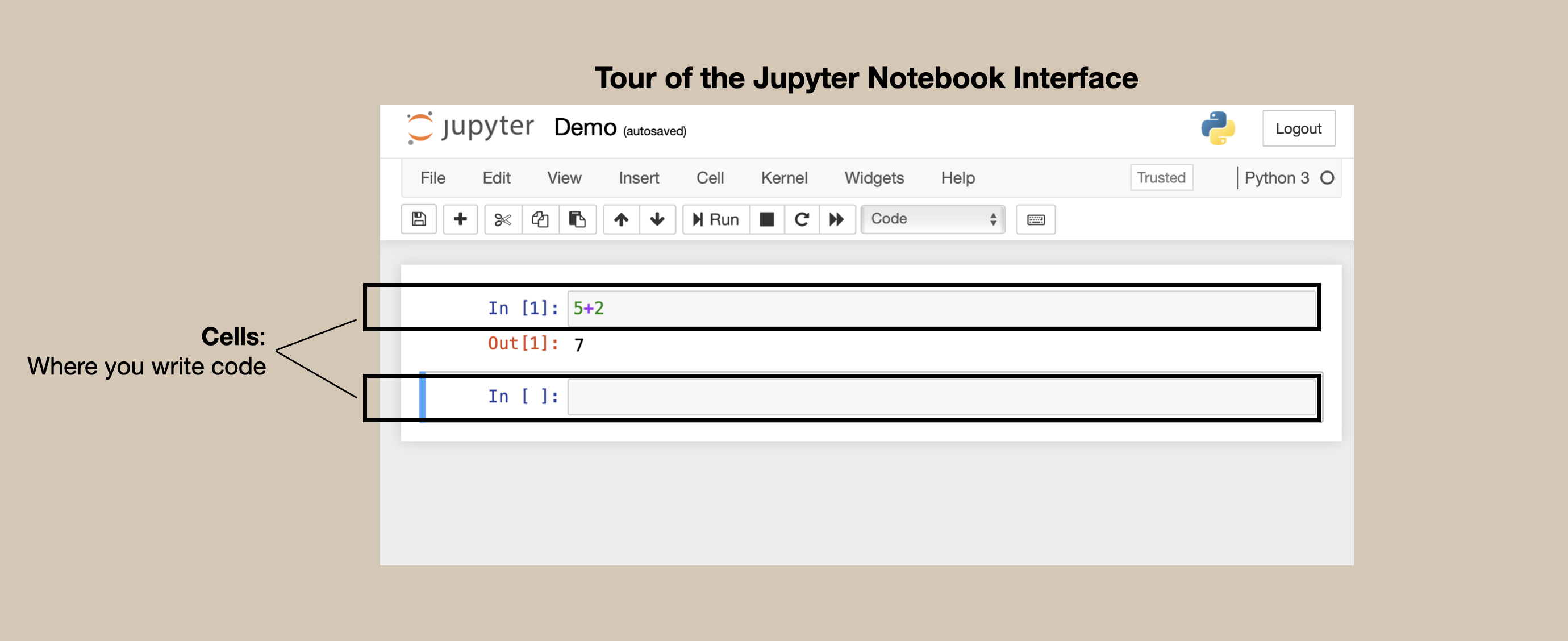
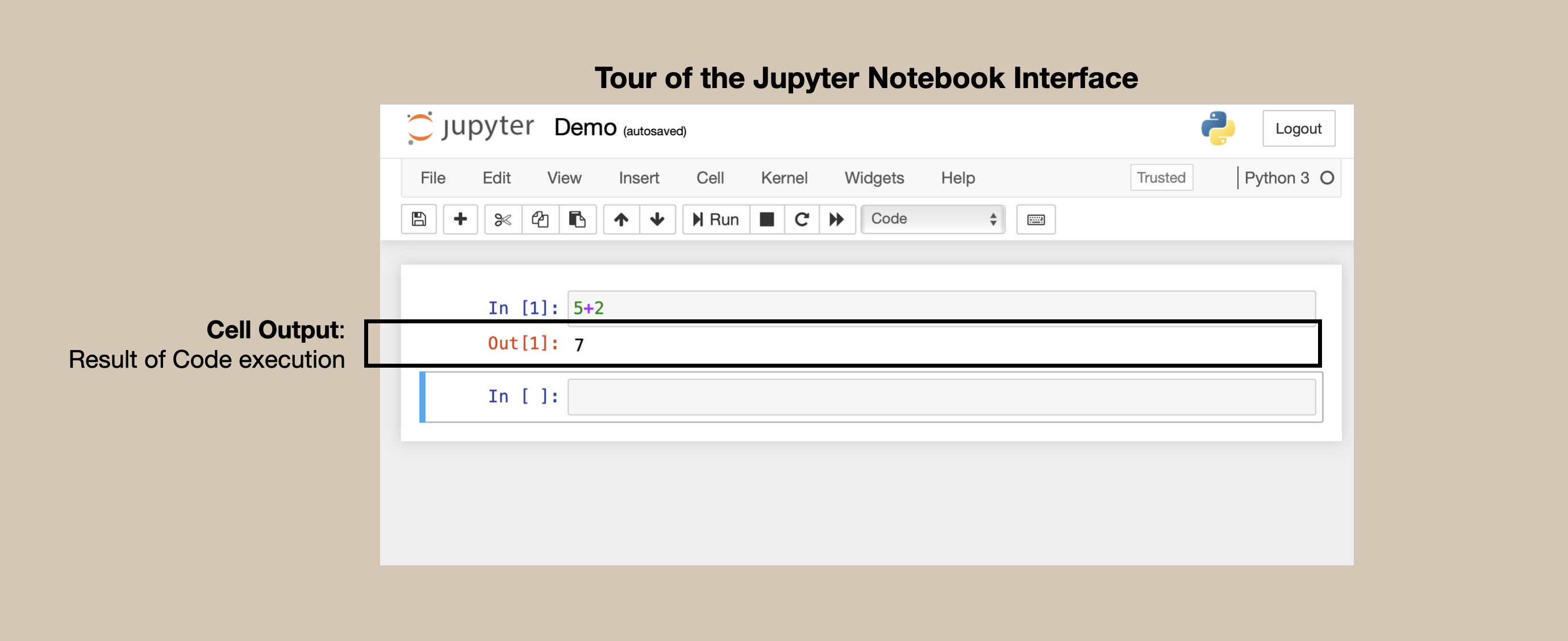
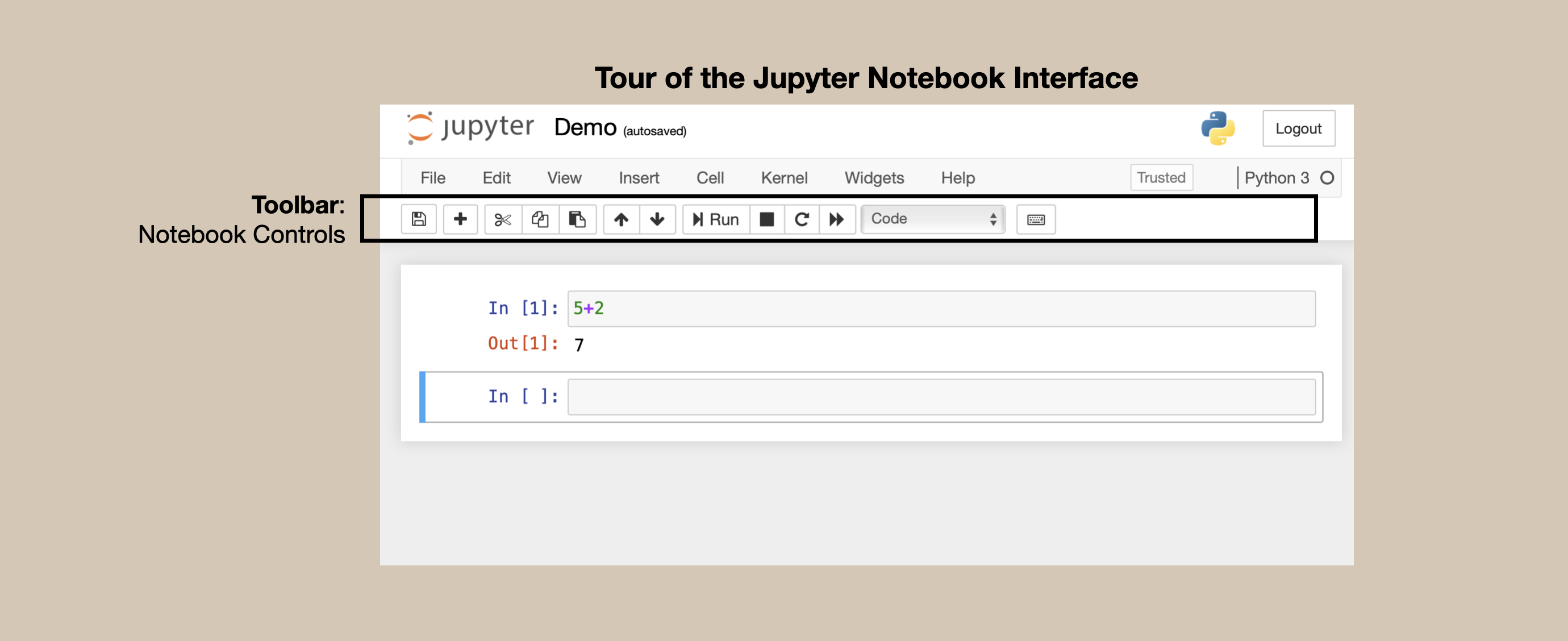
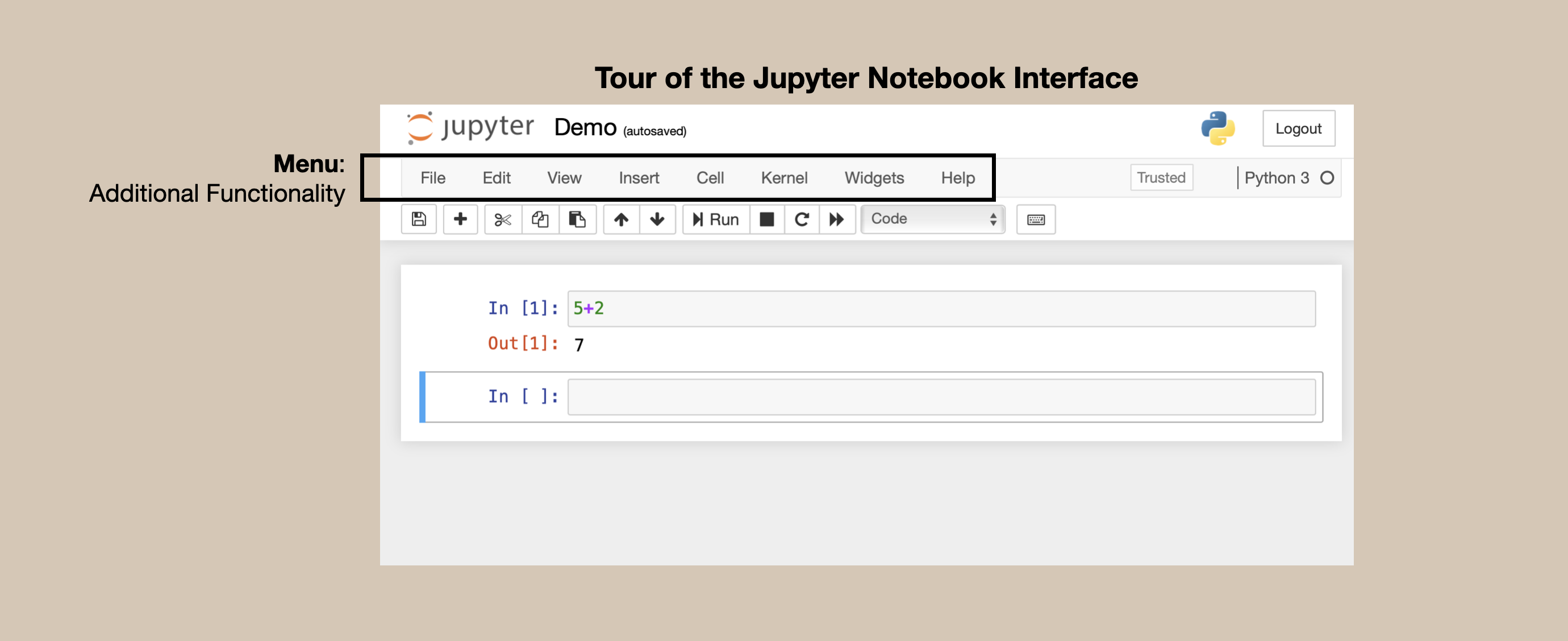
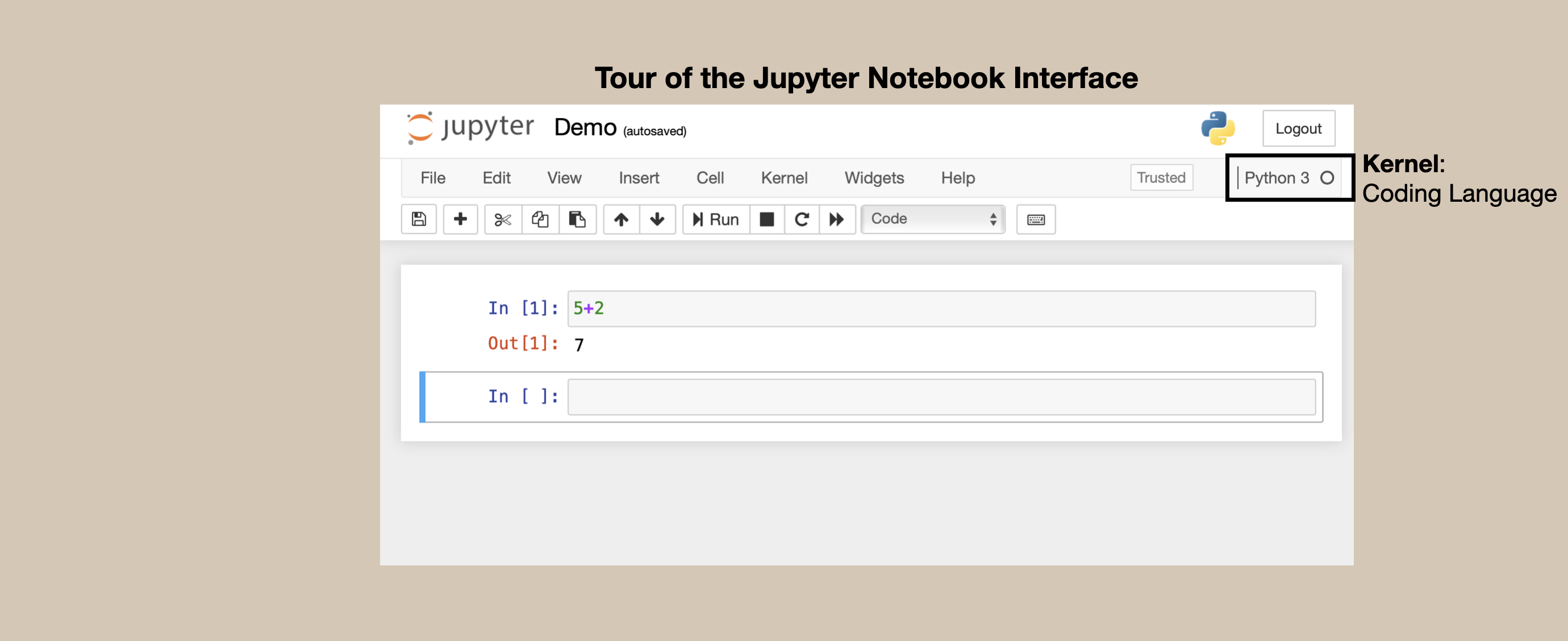
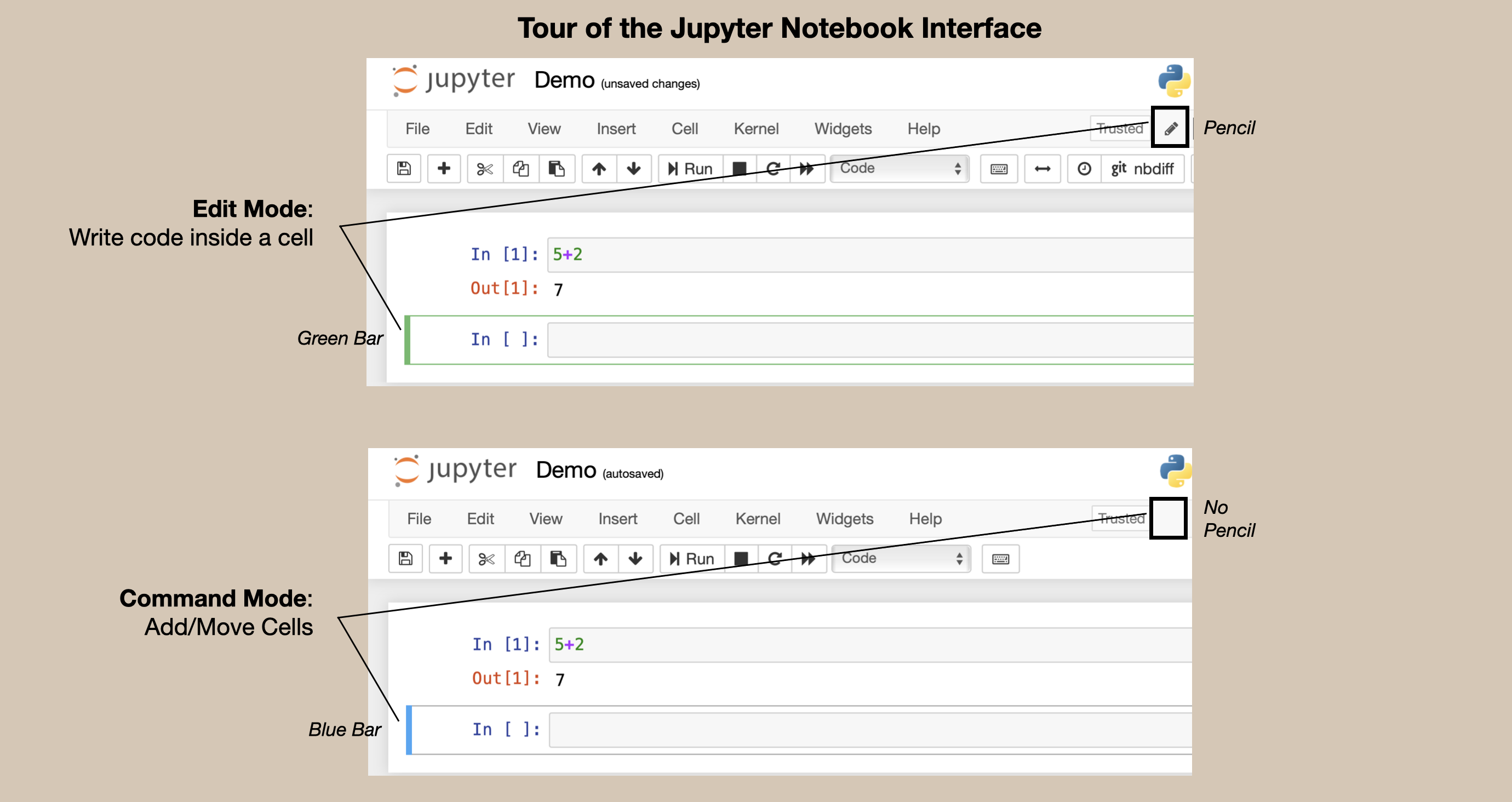
Useful things to know about JupyterLab#
Open JupyterLab
Create new notebooks
Entering and exiting “Command mode”
Converting cells to “markdown format”
Using JupyterLab for Markdown files
Close JupyterLab
Part 2: Writing and Running code (15 mins)#
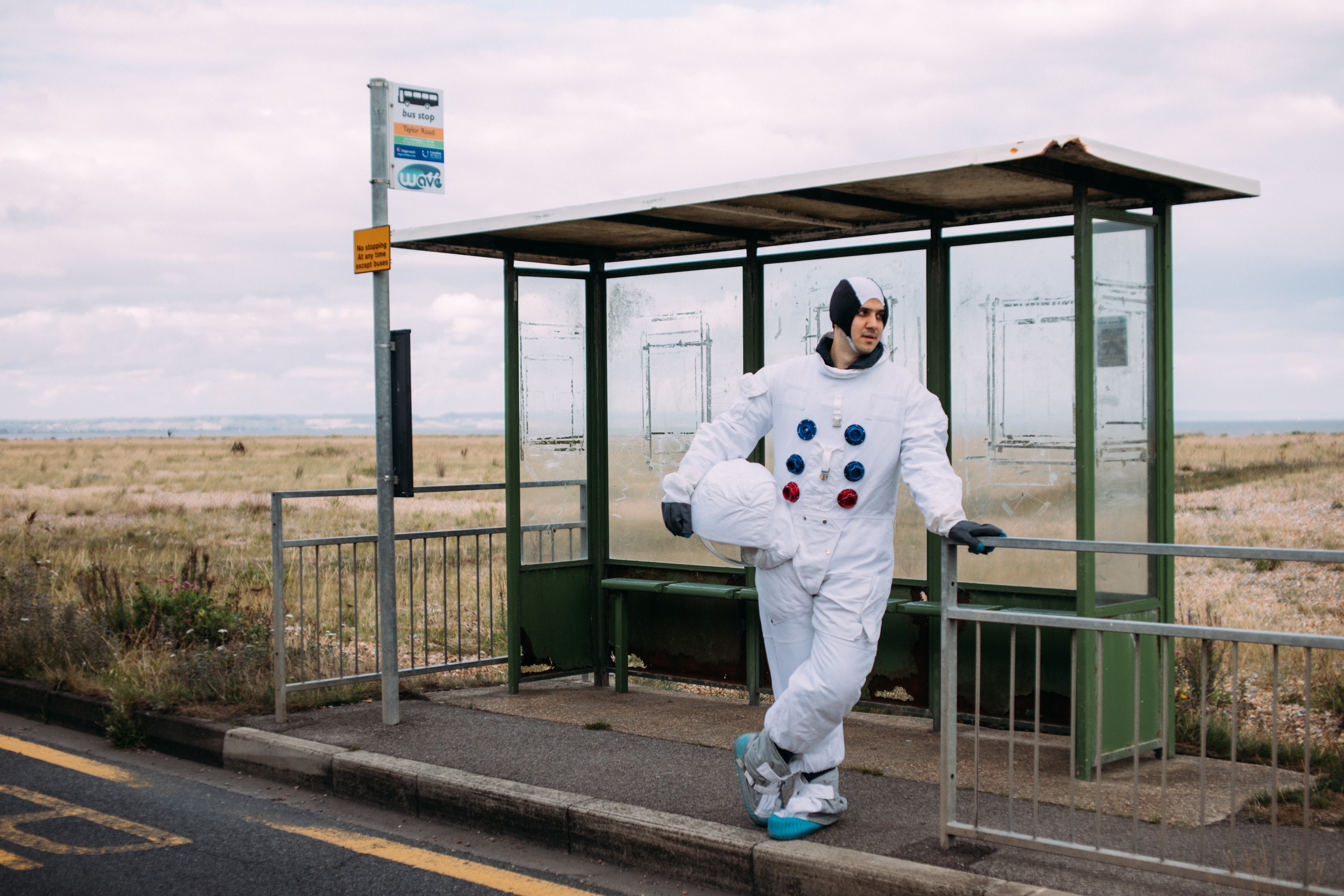
Using Python to do Math#
Task |
Symbol |
---|---|
Addition |
|
Subtraction |
|
Multiplication |
|
Division |
|
Square, cube, … |
|
Squareroot, Cuberoot, … |
|
Trigonometry (sin,cos,tan) |
Later… |
Demo (side-by-side)#
Addition#
4 + 5 + 2
11
# Subtraction
4 - 5
-1
# Multiplication
4 * 5
20
# Division
11 / 2
5.5
# Square, cube, ...
2**4
16
# Squareroot, Cuberoot, ...
27 ** (1 / 3)
3.0
Assigning numbers to variables#
You can assign numbers to a “variable”.
You can think of a variable as a “container” that represents what you assigned to it
There are some rules about “valid” names for variables, we’ll talk about the details later
Rule 1: Can’t start the name of a variable with a number!
General guideline for now: just use a combination of words and numbers
Demo (side-by-side)#
# Two numbers
num1 = 40
num2 = 12
print(num1, num2)
40 12
# Multiply numbers together
num1 * num2
480
Assigning words to variables#
You can also assign words and sentences to variables!
Surround anything that’s not a number with double quotes ” and “
mysentence = (
"This is a whole sentence with a list of sports, swimming, tennis, badminton"
)
print(mysentence)
This is a whole sentence with a list of sports, swimming, tennis, badminton
Using Python to work with words#
Python has some nifty “functions” to work with words and sentences.
Here’s a table summarizing some interesting ones, we’ll keep adding to this as the term goes on.
Task |
Function |
---|---|
Make everything upper-case |
|
Make everything lower-case |
|
Capitalize first letter of every word |
|
Count letters or sequences |
|
Demo (side-by-side)#
mysentence
'This is a whole sentence with a list of sports, swimming, tennis, badminton'
# make lower case
mysentence.lower()
'this is a whole sentence with a list of sports, swimming, tennis, badminton'
# make upper case
mysentence.upper()
'THIS IS A WHOLE SENTENCE WITH A LIST OF SPORTS, SWIMMING, TENNIS, BADMINTON'
# split on a comma
mysentence.split(",")
['This is a whole sentence with a list of sports',
' swimming',
' tennis',
' badminton']
mysentence.title()
'This Is A Whole Sentence With A List Of Sports, Swimming, Tennis, Badminton'
mysentence.upper().lower().upper().lower()
'this is a whole sentence with a list of sports, swimming, tennis, badminton'
# count the times "i" occurs
mysentence.count("with")
1
# count two characters: hi
mysentence.count("hi")
1
mysentence2 = " Hello. World ..... "
mysentence2
' Hello. World ..... '
mysentence2.strip(" ")
'Hello. World .....'
mysentence2.replace(" ", "^")
'^^^^^Hello.^^^^^World^.....^^^^^'
## RISE settings
from IPython.display import IFrame
from traitlets.config.manager import BaseJSONConfigManager
from pathlib import Path
path = Path.home() / ".jupyter" / "nbconfig"
cm = BaseJSONConfigManager(config_dir=str(path))
tmp = cm.update(
"rise",
{
"theme": "sky", # blood is dark, nice
"transition": "fade",
"start_slideshow_at": "selected",
"autolaunch": False,
"width": "100%",
"height": "100%",
"header": "",
"footer": "",
"scroll": True,
"enable_chalkboard": True,
"slideNumber": True,
"center": False,
"controlsLayout": "edges",
"slideNumber": True,
"hash": True,
},
)