Lecture 4 - Python¶
Slide formats:
Why learn Python?¶
Python is increasingly the most popular choice of programming language for data analysts because it is designed to be simple, efficient, and easy to read and write. There are many open source software and libraries that use Python and data analysis tools built on them. We will use Python to learn programming and explore fundamental programming concepts of commands, variables, decisions, repetition, and events.
What is Python?¶
Python is a general, high-level programming language designed for code readability and simplicity. Python is available for free as open source and has a large community supporting its development and associated tools. Python was developed by Guido van Rossum and first released in 1991. Python 2.0 was released in 2000 (latest version 2.7), and a backwards-incompatible release Python 3 was in 2008.
- Our coding style will be Python 3 but most code will also work for Python 2. - (P.S. the name comes from Monty Python)
Python Language Characteristics¶
Python supports:¶
dynamic typing - types can change at run-time
multi-paradigm – flexible in that is supports both procedural, object-oriented, and functional styles, for example.
automatic memory management and garbage collection
extensible – other languages such as C/C++ can be used to compile the code.
and more …
Python Language Characteristics¶
Python core philosophies (by Tim Peters)
Beautiful is better than ugly
Explicit is better than implicit
Simple is better than complex
Complex is better than complicated Readability counts
Some Quotes¶
If you can’t write it down in English, you can’t code it.
Peter Halpern I really hate this damn machine. I wish that I could sell it. I never does quite what I want. Just only what I tell it.
programmers lament
Introduction to Programming¶
Recall…
An algorithm is a precise sequence of steps to produce a result. A program is an encoding of an algorithm in a language to solve a particular problem.
There are numerous languages that programmers can use to specify instructions. Each language has its different features, benefits, and usefulness.
The goal is to understand fundamental programming concepts that apply to all languages.
Python: Basic Rules¶
To program in Python you must follow a set of rules for specifying your commands. This set of rules is called a syntax.
Just like any other language, there are rules that you must follow if you are to communicate correctly and precisely.
For a more thorough overview of Python, you may have a look at Python Essential Reference by David Beazleyas well as countless website online, eg w3schools, codeacademy, …
Other useful resources include: documentation for Python 3.7.3,Python.org (getting started), and the Pep 8 style guide.
Python: Basic Rules¶
Important general rules of Python syntax:
Python is case-sensitive.
Python is particular on whitespace and indentation.
The end of command is the end of line (i.e semi-colons are not a required terminator).
Use four spaces for indentation whenever in a block.
Spaces are the preferred indentation method.
Tabs should be used solely to remain consistent with code that is already indented with tabs.
Python 3 disallows mixing tabs and spaces for indentation.
def spam():
eggs = 12
return eggs
print spam()
Comments¶
Comments are used by the programmer to document and explain the code. Comments are ignored by the computer. Type # before the comment and any characters to the end of line are ignored by the computer. Example:
# Single line comment
print (1) # Comment at end of line
1
Python Programming¶
A Python program, like a book, is read left to right and top to bottom. Each command is on its own line.
# Sample Python program
name = "Joe"
print("Hello")
print("Name: "+name)
Hello
Name: Joe
A user types in a Python program in a text editor (some examples) or integrated development environment (IDE examples). To run the program we need a Python interpreter (i.e. a program that reads Python programs and carries out their instructions).
Installing and Using Python¶
You may already have a version of python on your computer. You can check by opening the command window/terminal and typing py/python (Windows/Mac). To exit using Python in the console type Ctrl + keystrokeZ then ENTER (if using Windows) or Ctrl + keystrokeD on a Mac. Alternatively, you could also run the python command exit(). Read more about running python through the console here. You could also use one of the various online Python 3 compilers for example.
Installing and Using Python¶
Python version
If you haven’t done any of the installations above, this is probably going to be Python 2.
You can find out which version of Python you have by typing python -V in your console.
Python Editor - Jupyter¶
“Project Jupyter exists to develop open-source software, open-standards, and services for interactive computing across dozens of programming languages.”
Jupyter notebook is a graphical, browser-based application for editing and running Python. Follow the instructions here to install.
To run, type jupyter notebook in your Command Prompt.
This action will start the notebook server in your default browser and echo information about the notebook server in your terminal. Read more about it by clicking Try Jupyter for Python here
Python Editor - Jupyter¶
The Notebook Dashboard will list all of the notebooks (.ipynb), files, and subdirectories stored in the local working directory (i.e. the directory from which you launched jupyter)
Python Editor - Jupyter¶
To close this program, navigate the the Running tab and click the orange Shutdown button. Closing the notebook’s page is not sufficient to shutdown.
Python Editor - Jupyter¶
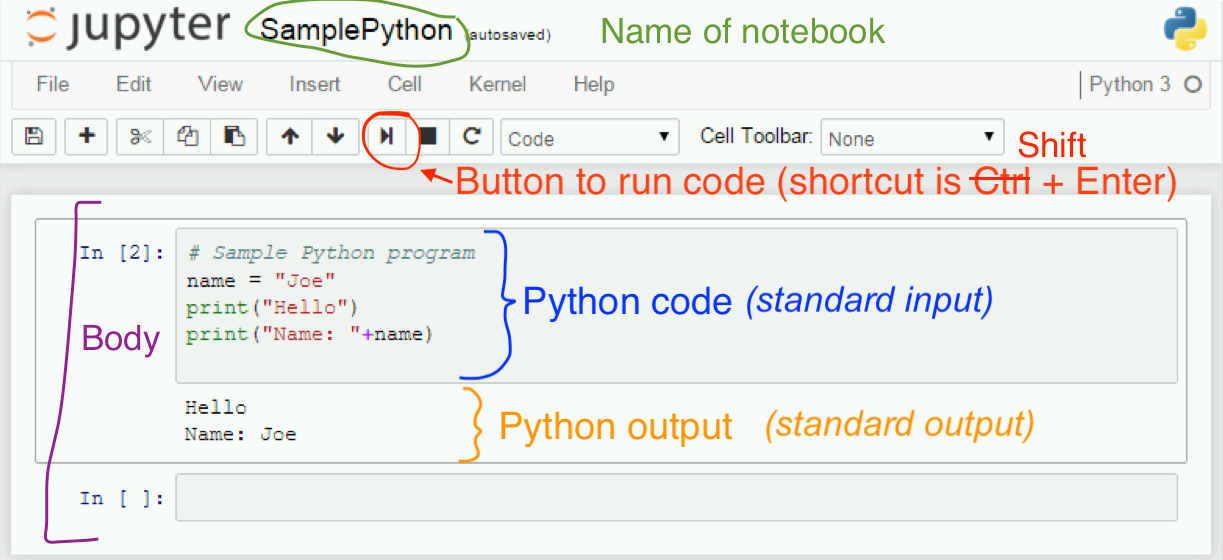
Python Editor – jupyter notebook¶
The body of the notbook is comprised of cells:
Markdown cells are used to build write regular (non-code) text. More markdown here and see helpful cheatsheet.
Code cells (default) are used to define the code which will be compiled (after pressing or pressing Shift + Enter ) to produce output.
You can inserts cells (either code/markdown) anywhere by clicking Insert > Insert Cell Above (or Insert Cell Below)
Same thing goes for deleting/copying/pasting/undoing cells: see the Edit drop down menu bar.
Python Editor – jupyter notebook¶
You can create regular text and headings in markdown mode. Headings are indicated using # (subheadings use ##)
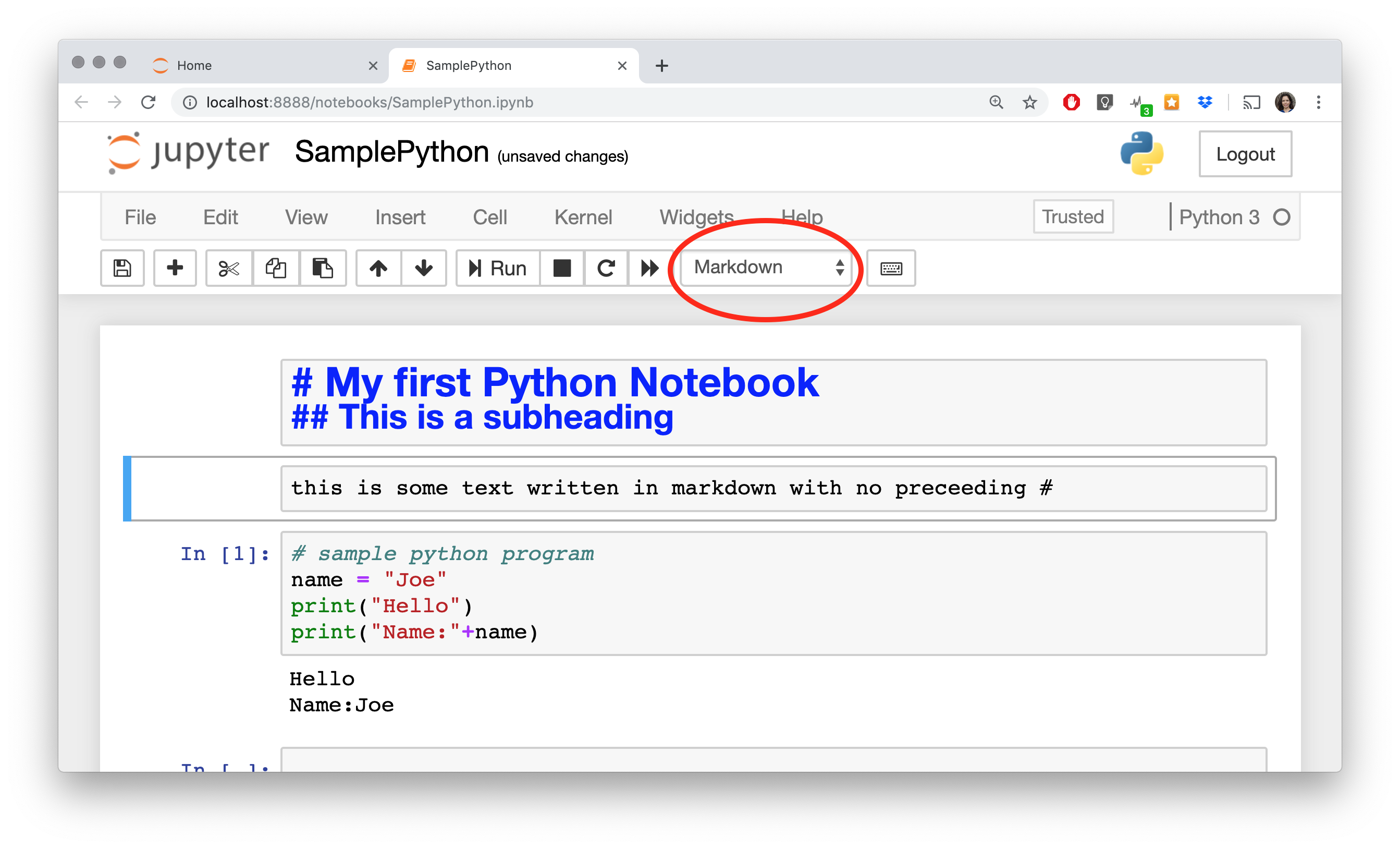
Python Editor – jupyter notebook¶
After a cell is run, a number will appear in the square parenthesis (an asterisk * will appear for cells that are currently running). Every time we run that cell, this number will increase.
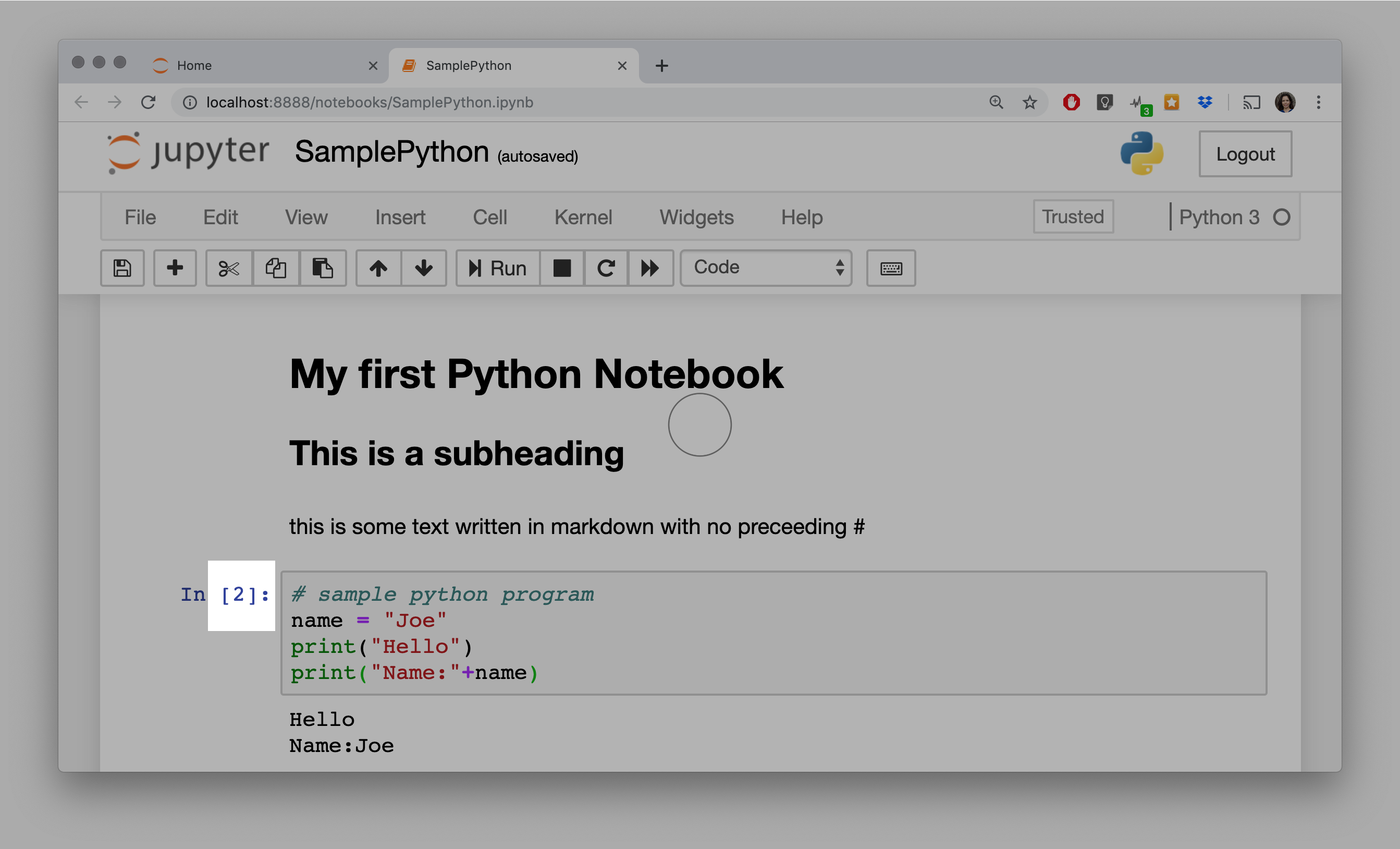
Python Editor – jupyter notebook¶
While jupyter notebook does autosave periodically, it is a good to save your work upon exiting (go to File > Save and Checkpoint or click the save icon).
When we open the file again, it is not guaranteed that everything we need is in memory.
It is good practice to “reset” the session by clicking Kernel > Restart and Run all Cells to ensure everything we need has been loaded into our working session (note that this will reset all of our numbers in the square brackets).
Python: Hello World!¶
A traditional introduction into programming involves outputting the message “Hello World!” In Python3, this is a simple as typing:
print("Hello World!")
Hello World!
The print function will print to the terminal (standard output) whatever data (number, string, variable) it is given.
You can use double quotes (“Hello world”) or single quotes (‘Hello world’) just be consistent! E.g. ‘Hello World!” will produce a error.
Tripple Quotes¶
If your message runs across multiple lines, you can use 3 quotations to denote multi-line strings.
The sting begins with a ‘’’ (or “””) and ends with a (or “””).
Note that in this environment, we can use linebreaks (ie we can start a new line by pressing ENTER) and include the single and double quotes with our string.
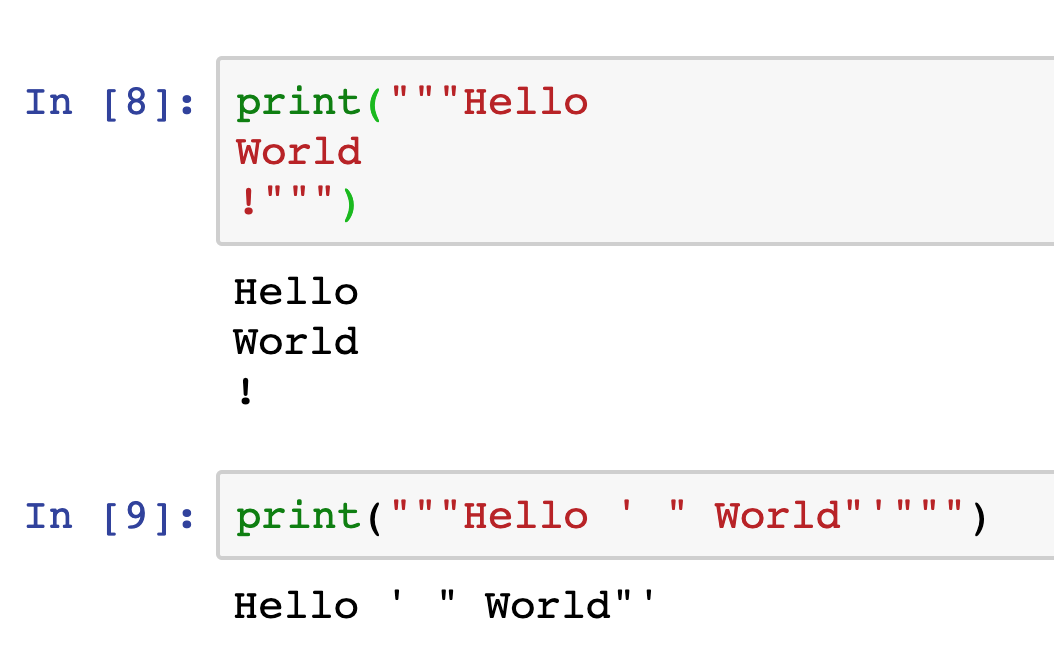
Try It: Python Printing¶
Example 1
Write a Python program that prints "I can start coding!"
Example 2
Write a Python program that prints these three lines:
I know that I can program in Python. I am programming right now.
My awesome program has three lines!
Example 3
How many of the following statements are TRUE?
1. Python is case-sensitive.
2. A command in Python must be terminated by a semi-colon. 3. Indentation does not matter in Python.
4. A single line comment starts with """.
5. The print command prints to standard input.
A) 0 B) 1 C) 2 D) 3 E) 4
Answer:
How many of the following statements are TRUE?
1. Python is case-sensitive.
2. A command in Python must be terminated by a semi-colon. 3. Indentation does not matter in Python.
4. A single line comment starts with """"".
5. The print command prints to standard input.
A) 0 B) 1 C) 2 D) 3 E) 4
Answer:
How many of the following statements are TRUE?
1. Python is case-sensitive.
2. A command in Python must be terminated by a semi-colon. 3. Indentation does not matter in Python.
4. A single line comment starts with """"".
5. The print command prints to standard input.
A) 0 B) 1 C) 2 D) 3 E) 4
Variables¶
Recall… A variable is a name that refers to a location that stores a data value. IMPORTANT: The value at a location can change using initialization or assignment.
Variable Assignment¶
In python the assignment operator is “=”. We will use it to (re)set value of a variable.
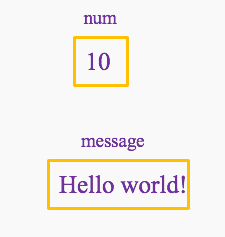
# Example:
num = 10
message = "Hello world!"
Python Variables¶
Variables are created when first assigned.
A variable type is dynamic and do not need to be declared.
It can store any particular type (e.g. int, float, strings, or Boolean) at any given time.
# Example:
val = 5
val = "Hello"
isAwesome = True
Recall: Boolean values can be either True or False. N.B. in Python case matters. The type (string, int, float etc.) of the variable is determined by Python.
Variable Rules¶
Variables are a name that must begin with a letter or an underscore and cannot contain spaces. All subsequent characters must be letters, numbers or underscores.
Variables are created when they are first used. There is no special syntax to declare (create) a variable.
Variable names are case-sensitive.
A programmer picks the names for variables, but try to make the names meaningful and explain their purpose.
Avoid naming variables as reserved words (e.g. if, for, else). A reserved word has special meaning in the language.
Example 4
Which of the following variable names are valid?
1. name
2. string2
3. 2cool
4. under_score
5. space name
6. else
End of Lecture 4 on September 30, 2020